你有没有想过,用Python和以太坊玩儿起来会是怎样的感觉?就像是拿着一把魔法钥匙,打开了通往区块链世界的神秘之门。别愣着了,今天就来带你一探究竟,看看Python是如何召唤出以太坊的神奇力量的!
一、Python的魔法棒:web3.py
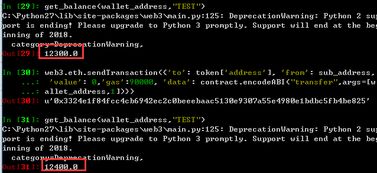
首先,你得有一根魔法棒,这根棒子就是web3.py。它是Python与以太坊之间沟通的桥梁,让你能够轻松地与区块链互动。想象你只需要几行代码,就能查询区块链上的信息,甚至还能发送交易,是不是很神奇?
安装与配置
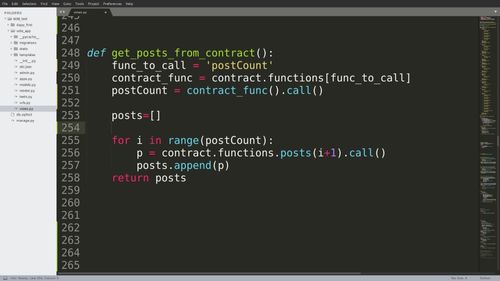
别急,先来准备一下你的魔法棒。打开你的终端,输入以下命令:
```bash
pip install web3
安装完成后,你就可以开始你的魔法之旅了。
二、连接到以太坊
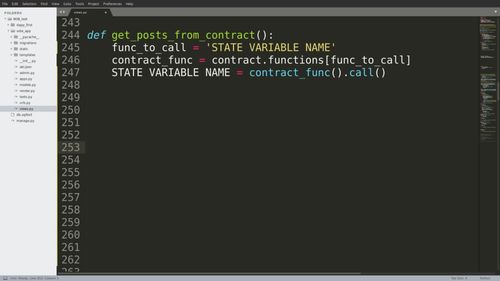
有了魔法棒,下一步就是找到你的以太坊节点。你可以选择连接到本地节点,比如Ganache,也可以连接到线上节点,比如Infura。这里以Ganache为例,它就像是一个私人的以太坊世界,非常适合测试和学习。
连接Ganache
```python
from web3 import Web3
连接到本地Ganache节点
web3 = Web3(Web3.HTTPProvider('http://localhost:8545'))
如果你选择连接到线上节点,只需将URL替换为相应的节点地址即可。
三、探索区块链
现在,你已经连接到了以太坊,可以开始探索这个神秘的世界了。比如,你可以查询最新的区块信息,看看最近都有哪些交易发生。
查询最新区块
```python
latest_block = web3.eth.get_block('latest')
print(latest_block)
怎么样,是不是很简单?你还可以查询某个特定区块的信息,或者获取某个账户的余额。
四、发送交易
如果你想要在以太坊上发送交易,比如转账,那就更简单了。你只需要准备一些交易参数,然后调用相应的函数即可。
发送交易
```python
准备交易参数
from_address = '你的以太坊地址'
to_address = '接收方的以太坊地址'
value = web3.toWei(1, 'ether') 转账金额,这里以1以太坊为例
构建交易
nonce = web3.eth.getTransactionCount(from_address)
gas = web3.toWei('21000', 'gwei') 交易费用
gas_price = web3.toWei('20', 'gwei') 气价
transaction = {
'nonce': nonce,
'gas': gas,
'gasPrice': gas_price,
'to': to_address,
'value': value,
签名交易
signed_txn = web3.eth.account.sign_transaction(transaction, private_key='你的私钥')
发送交易
tx_hash = web3.eth.sendRawTransaction(signed_txn.rawTransaction)
print(tx_hash.hex())
怎么样,是不是感觉像是在玩儿一个游戏?你只需要按照规则操作,就能在以太坊上完成交易。
五、智能合约:Python与Solidity的完美结合
当然,以太坊的魅力不仅仅在于转账,更在于智能合约。智能合约是一种自动执行的合约,它可以在区块链上执行各种复杂的操作。
编写智能合约
```solidity
// SimpleBank.sol
pragma solidity ^0.8.0;
contract SimpleBank {
mapping(address => uint) private balances;
// 存款
function deposit() public payable {
balances[msg.sender()] += msg.value;
}
// 取款
function withdraw() public {
uint balance = balances[msg.sender()];
require(balance > 0, \余额不足\);
balances[msg.sender()] -= balance;
payable(msg.sender()).transfer(balance);
}
部署智能合约
```python
from web3 import Web3
连接到以太坊节点
web3 = Web3(Web3.HTTPProvider('http://localhost:8545'))
编译智能合约
with open('SimpleBank.sol', 'r') as file:
contract_source = file.read()
compiled_sol = web3.compile(contract_source)
部署智能合约
contract = web3.eth.contract(abi=compiled_sol['SimpleBank.abi'], bytecode=compiled_sol['SimpleBank.bytecode'])
nonce = web3.eth.getTransactionCount('你的以太坊地址')
transaction = contract.constructor().buildTransaction({
'nonce': nonce,
'gas': 2000000,
'gasPrice': web3.toWei('50', 'gwei'),
signed_txn = web3.eth.account.sign_transaction(transaction, private_key='你的私钥')
tx_hash = web3.eth.sendRawTransaction(signed_txn.rawTransaction)
tx_receipt = web3.eth.waitForTransactionReceipt(tx_hash)
获取合约地址
contract_address